Aggregation processor
The aggregator processor will combine multiple mesh objects into one single object. The output geometry and each individual texture chart is identical to the inputs, but the charts have been combined and repositioned into a new, unified texture atlas. In relation to the usual Simplygon parameterizer, which generates new, unique UVs for every point on the mesh, the aggregator is a good solution for combining multiple instances of the same object, or when dealing with tiled textures. Multiple instances of an object will share the same texture coordinates once combined (if the separate overlapping charts settings is false).
The following figure shows the scene aggregator having been run on a scene with two identical half-sphere objects. The half-spheres shared UVs before processing, and continue to share UVs after processing and packing the charts into a new atlas.
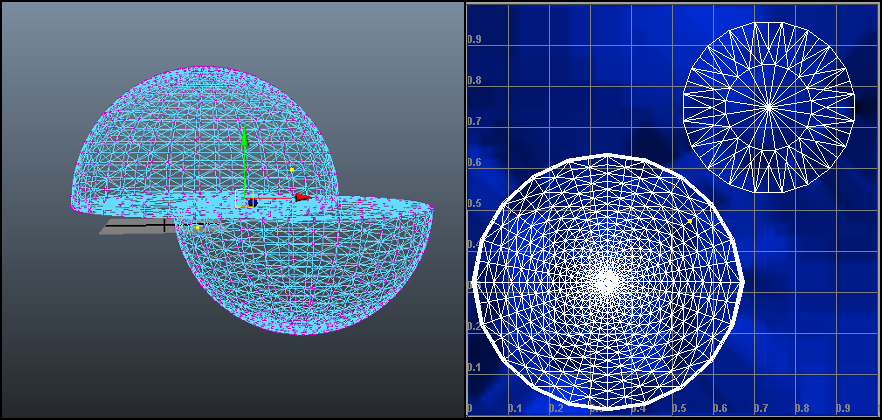
One good example when to use the scene aggregator is when you want to combine objects to define a building, where each window is an instance of each other. The texture coordinates and materials for the windows are the same and the texture atlas will only store one material for all the windows in the building. In this way you get a resulting material/texture with much better resolution, compared to the one generated by the standard parameterizer, which will have to store materials for every window object. In the figure above two half-sphere objects can be visualized, where one of them is an instance of the other. As their data (texture coordinates and material) is exactly the same, only one material need to be added to the final material/texture.
Figure | Description |
---|---|
![]() | A scene including twenty objects and three materials. |
![]() | Difference in the final material when using scene aggregator (right) or the standard parameterizer in the reducer (left).< |
Aggregation processor settings
This settings object contains the settings relevant for the scene aggregator. Since the main function of the scene aggregator is chart aggregation, most of the relevant settings are located in chart aggregator settings, which contains the controls for all parameterization.
Example
This example shows how to use the Aggregation processor.
// Copyright (c) Microsoft Corporation.
// Licensed under the MIT License.
#include <string>
#include <stdlib.h>
#include <filesystem>
#include <future>
#include "SimplygonLoader.h"
Simplygon::spScene LoadScene(Simplygon::ISimplygon* sg, const char* path)
{
// Create scene importer
Simplygon::spSceneImporter sgSceneImporter = sg->CreateSceneImporter();
sgSceneImporter->SetImportFilePath(path);
// Run scene importer.
auto importResult = sgSceneImporter->Run();
if (Simplygon::Failed(importResult))
{
throw std::exception("Failed to load scene.");
}
Simplygon::spScene sgScene = sgSceneImporter->GetScene();
return sgScene;
}
void SaveScene(Simplygon::ISimplygon* sg, Simplygon::spScene sgScene, const char* path)
{
// Create scene exporter.
Simplygon::spSceneExporter sgSceneExporter = sg->CreateSceneExporter();
std::string outputScenePath = std::string("output\\") + std::string("Aggregation") + std::string("_") + std::string(path);
sgSceneExporter->SetExportFilePath(outputScenePath.c_str());
sgSceneExporter->SetScene(sgScene);
// Run scene exporter.
auto exportResult = sgSceneExporter->Run();
if (Simplygon::Failed(exportResult))
{
throw std::exception("Failed to save scene.");
}
}
void CheckLog(Simplygon::ISimplygon* sg)
{
// Check if any errors occurred.
bool hasErrors = sg->ErrorOccurred();
if (hasErrors)
{
Simplygon::spStringArray errors = sg->CreateStringArray();
sg->GetErrorMessages(errors);
auto errorCount = errors->GetItemCount();
if (errorCount > 0)
{
printf("%s\n", "CheckLog: Errors:");
for (auto errorIndex = 0U; errorIndex < errorCount; ++errorIndex)
{
Simplygon::spString errorString = errors->GetItem((int)errorIndex);
printf("%s\n", errorString.c_str());
}
sg->ClearErrorMessages();
}
}
else
{
printf("%s\n", "CheckLog: No errors.");
}
// Check if any warnings occurred.
bool hasWarnings = sg->WarningOccurred();
if (hasWarnings)
{
Simplygon::spStringArray warnings = sg->CreateStringArray();
sg->GetWarningMessages(warnings);
auto warningCount = warnings->GetItemCount();
if (warningCount > 0)
{
printf("%s\n", "CheckLog: Warnings:");
for (auto warningIndex = 0U; warningIndex < warningCount; ++warningIndex)
{
Simplygon::spString warningString = warnings->GetItem((int)warningIndex);
printf("%s\n", warningString.c_str());
}
sg->ClearWarningMessages();
}
}
else
{
printf("%s\n", "CheckLog: No warnings.");
}
// Error out if Simplygon has errors.
if (hasErrors)
{
throw std::exception("Processing failed with an error");
}
}
void RunAggregation(Simplygon::ISimplygon* sg)
{
// Load scene to process.
printf("%s\n", "Load scene to process.");
Simplygon::spScene sgScene = LoadScene(sg, "../../../Assets/SimplygonMan/SimplygonMan.obj");
// Create the aggregation processor.
Simplygon::spAggregationProcessor sgAggregationProcessor = sg->CreateAggregationProcessor();
sgAggregationProcessor->SetScene( sgScene );
Simplygon::spAggregationSettings sgAggregationSettings = sgAggregationProcessor->GetAggregationSettings();
// Merge all geometries into a single geometry.
sgAggregationSettings->SetMergeGeometries( true );
// Start the aggregation process.
printf("%s\n", "Start the aggregation process.");
sgAggregationProcessor->RunProcessing();
// Save processed scene.
printf("%s\n", "Save processed scene.");
SaveScene(sg, sgScene, "Output.fbx");
// Check log for any warnings or errors.
printf("%s\n", "Check log for any warnings or errors.");
CheckLog(sg);
}
int main()
{
Simplygon::ISimplygon* sg = NULL;
Simplygon::EErrorCodes initval = Simplygon::Initialize( &sg );
if( initval != Simplygon::EErrorCodes::NoError )
{
printf( "Failed to initialize Simplygon: ErrorCode(%d)", (int)initval );
return int(initval);
}
RunAggregation(sg);
Simplygon::Deinitialize(sg);
return 0;
}
// Copyright (c) Microsoft Corporation.
// Licensed under the MIT License.
using System;
using System.IO;
using System.Threading.Tasks;
public class Program
{
static Simplygon.spScene LoadScene(Simplygon.ISimplygon sg, string path)
{
// Create scene importer
using Simplygon.spSceneImporter sgSceneImporter = sg.CreateSceneImporter();
sgSceneImporter.SetImportFilePath(path);
// Run scene importer.
var importResult = sgSceneImporter.Run();
if (Simplygon.Simplygon.Failed(importResult))
{
throw new System.Exception("Failed to load scene.");
}
Simplygon.spScene sgScene = sgSceneImporter.GetScene();
return sgScene;
}
static void SaveScene(Simplygon.ISimplygon sg, Simplygon.spScene sgScene, string path)
{
// Create scene exporter.
using Simplygon.spSceneExporter sgSceneExporter = sg.CreateSceneExporter();
string outputScenePath = string.Join("", new string[] { "output\\", "Aggregation", "_", path });
sgSceneExporter.SetExportFilePath(outputScenePath);
sgSceneExporter.SetScene(sgScene);
// Run scene exporter.
var exportResult = sgSceneExporter.Run();
if (Simplygon.Simplygon.Failed(exportResult))
{
throw new System.Exception("Failed to save scene.");
}
}
static void CheckLog(Simplygon.ISimplygon sg)
{
// Check if any errors occurred.
bool hasErrors = sg.ErrorOccurred();
if (hasErrors)
{
Simplygon.spStringArray errors = sg.CreateStringArray();
sg.GetErrorMessages(errors);
var errorCount = errors.GetItemCount();
if (errorCount > 0)
{
Console.WriteLine("CheckLog: Errors:");
for (uint errorIndex = 0; errorIndex < errorCount; ++errorIndex)
{
string errorString = errors.GetItem((int)errorIndex);
Console.WriteLine(errorString);
}
sg.ClearErrorMessages();
}
}
else
{
Console.WriteLine("CheckLog: No errors.");
}
// Check if any warnings occurred.
bool hasWarnings = sg.WarningOccurred();
if (hasWarnings)
{
Simplygon.spStringArray warnings = sg.CreateStringArray();
sg.GetWarningMessages(warnings);
var warningCount = warnings.GetItemCount();
if (warningCount > 0)
{
Console.WriteLine("CheckLog: Warnings:");
for (uint warningIndex = 0; warningIndex < warningCount; ++warningIndex)
{
string warningString = warnings.GetItem((int)warningIndex);
Console.WriteLine(warningString);
}
sg.ClearWarningMessages();
}
}
else
{
Console.WriteLine("CheckLog: No warnings.");
}
// Error out if Simplygon has errors.
if (hasErrors)
{
throw new System.Exception("Processing failed with an error");
}
}
static void RunAggregation(Simplygon.ISimplygon sg)
{
// Load scene to process.
Console.WriteLine("Load scene to process.");
Simplygon.spScene sgScene = LoadScene(sg, "../../../Assets/SimplygonMan/SimplygonMan.obj");
// Create the aggregation processor.
using Simplygon.spAggregationProcessor sgAggregationProcessor = sg.CreateAggregationProcessor();
sgAggregationProcessor.SetScene( sgScene );
using Simplygon.spAggregationSettings sgAggregationSettings = sgAggregationProcessor.GetAggregationSettings();
// Merge all geometries into a single geometry.
sgAggregationSettings.SetMergeGeometries( true );
// Start the aggregation process.
Console.WriteLine("Start the aggregation process.");
sgAggregationProcessor.RunProcessing();
// Save processed scene.
Console.WriteLine("Save processed scene.");
SaveScene(sg, sgScene, "Output.fbx");
// Check log for any warnings or errors.
Console.WriteLine("Check log for any warnings or errors.");
CheckLog(sg);
}
static int Main(string[] args)
{
using var sg = Simplygon.Loader.InitSimplygon(out var errorCode, out var errorMessage);
if (errorCode != Simplygon.EErrorCodes.NoError)
{
Console.WriteLine( $"Failed to initialize Simplygon: ErrorCode({(int)errorCode}) {errorMessage}" );
return (int)errorCode;
}
RunAggregation(sg);
return 0;
}
}
# Copyright (c) Microsoft Corporation.
# Licensed under the MIT License.
import math
import os
import sys
import glob
import gc
import threading
from pathlib import Path
from simplygon10 import simplygon_loader
from simplygon10 import Simplygon
def LoadScene(sg: Simplygon.ISimplygon, path: str):
# Create scene importer
sgSceneImporter = sg.CreateSceneImporter()
sgSceneImporter.SetImportFilePath(path)
# Run scene importer.
importResult = sgSceneImporter.Run()
if Simplygon.Failed(importResult):
raise Exception('Failed to load scene.')
sgScene = sgSceneImporter.GetScene()
return sgScene
def SaveScene(sg: Simplygon.ISimplygon, sgScene: Simplygon.spScene, path: str):
# Create scene exporter.
sgSceneExporter = sg.CreateSceneExporter()
outputScenePath = ''.join(['output\\', 'Aggregation', '_', path])
sgSceneExporter.SetExportFilePath(outputScenePath)
sgSceneExporter.SetScene(sgScene)
# Run scene exporter.
exportResult = sgSceneExporter.Run()
if Simplygon.Failed(exportResult):
raise Exception('Failed to save scene.')
def CheckLog(sg: Simplygon.ISimplygon):
# Check if any errors occurred.
hasErrors = sg.ErrorOccurred()
if hasErrors:
errors = sg.CreateStringArray()
sg.GetErrorMessages(errors)
errorCount = errors.GetItemCount()
if errorCount > 0:
print('CheckLog: Errors:')
for errorIndex in range(errorCount):
errorString = errors.GetItem(errorIndex)
print(errorString)
sg.ClearErrorMessages()
else:
print('CheckLog: No errors.')
# Check if any warnings occurred.
hasWarnings = sg.WarningOccurred()
if hasWarnings:
warnings = sg.CreateStringArray()
sg.GetWarningMessages(warnings)
warningCount = warnings.GetItemCount()
if warningCount > 0:
print('CheckLog: Warnings:')
for warningIndex in range(warningCount):
warningString = warnings.GetItem(warningIndex)
print(warningString)
sg.ClearWarningMessages()
else:
print('CheckLog: No warnings.')
# Error out if Simplygon has errors.
if hasErrors:
raise Exception('Processing failed with an error')
def RunAggregation(sg: Simplygon.ISimplygon):
# Load scene to process.
print("Load scene to process.")
sgScene = LoadScene(sg, '../../../Assets/SimplygonMan/SimplygonMan.obj')
# Create the aggregation processor.
sgAggregationProcessor = sg.CreateAggregationProcessor()
sgAggregationProcessor.SetScene( sgScene )
sgAggregationSettings = sgAggregationProcessor.GetAggregationSettings()
# Merge all geometries into a single geometry.
sgAggregationSettings.SetMergeGeometries( True )
# Start the aggregation process.
print("Start the aggregation process.")
sgAggregationProcessor.RunProcessing()
# Save processed scene.
print("Save processed scene.")
SaveScene(sg, sgScene, 'Output.fbx')
# Check log for any warnings or errors.
print("Check log for any warnings or errors.")
CheckLog(sg)
if __name__ == '__main__':
sg = simplygon_loader.init_simplygon()
if sg is None:
exit(Simplygon.GetLastInitializationError())
RunAggregation(sg)
sg = None
gc.collect()