Getting started - Scripting
Simplygon will start the optimization once the Simplygon command is executed in combination with a Pipeline object or a Pipeline file-path. Execute either the MEL- or Python version of the script by pressing the double-arrow in the script windows's menu-bar to start the processing, the optimized scene will be returned to Maya automatically once the processing has completed.
MEL script
// select objects in scene
select -all;
// create a reduction Pipeline using the SimplygonPipeline command
$reductionPipeline = `SimplygonPipeline -c "ReductionPipeline"`;
// set the reduction Pipeline's triangle ratio to 50%
$bResult = `SimplygonPipeline -ss "ReductionProcessor/ReductionSettings/ReductionTargetTriangleRatio" -v 0.5 $reductionPipeline`;
// execute Simplygon with the given Pipeline
Simplygon -so $reductionPipeline;
// clear all loaded / created Pipelines
SimplygonPipeline -cl;
Maya script window - MEL
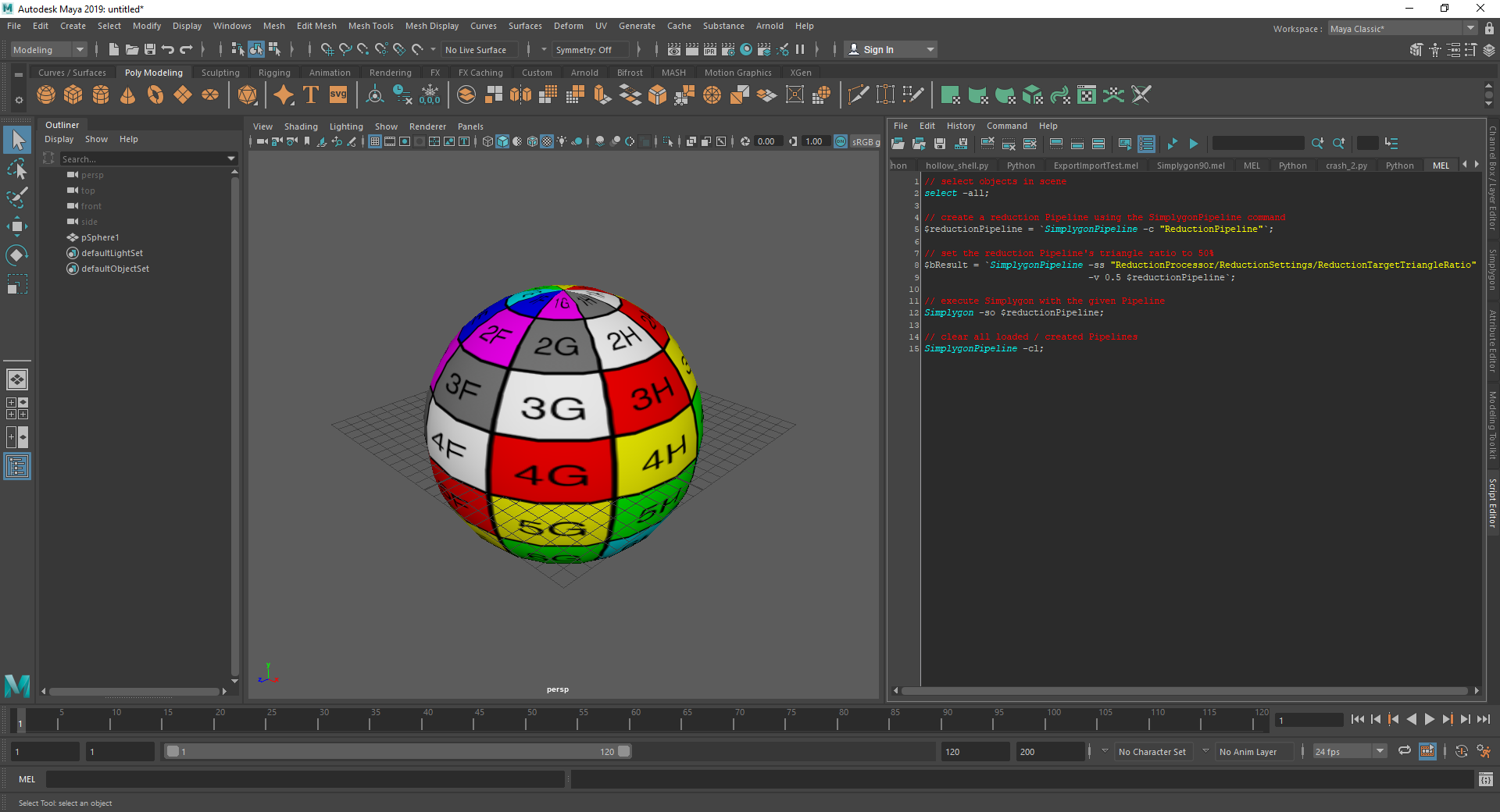
Python script
import maya.cmds as cmds
# select objects in scene
cmds.select(all = True)
# create a reduction Pipeline using the SimplygonPipeline command
reductionPipeline = cmds.SimplygonPipeline(c = 'ReductionPipeline')
# set the reduction Pipeline's triangle ratio to 50%
bResult = cmds.SimplygonPipeline(reductionPipeline, ss = 'ReductionProcessor/ReductionSettings/ReductionTargetTriangleRatio', v = 0.5)
# execute Simplygon with the given Pipeline
cmds.Simplygon(so = reductionPipeline)
# clear all loaded / created Pipelines
cmds.SimplygonPipeline(cl = True)
Maya script window - Python
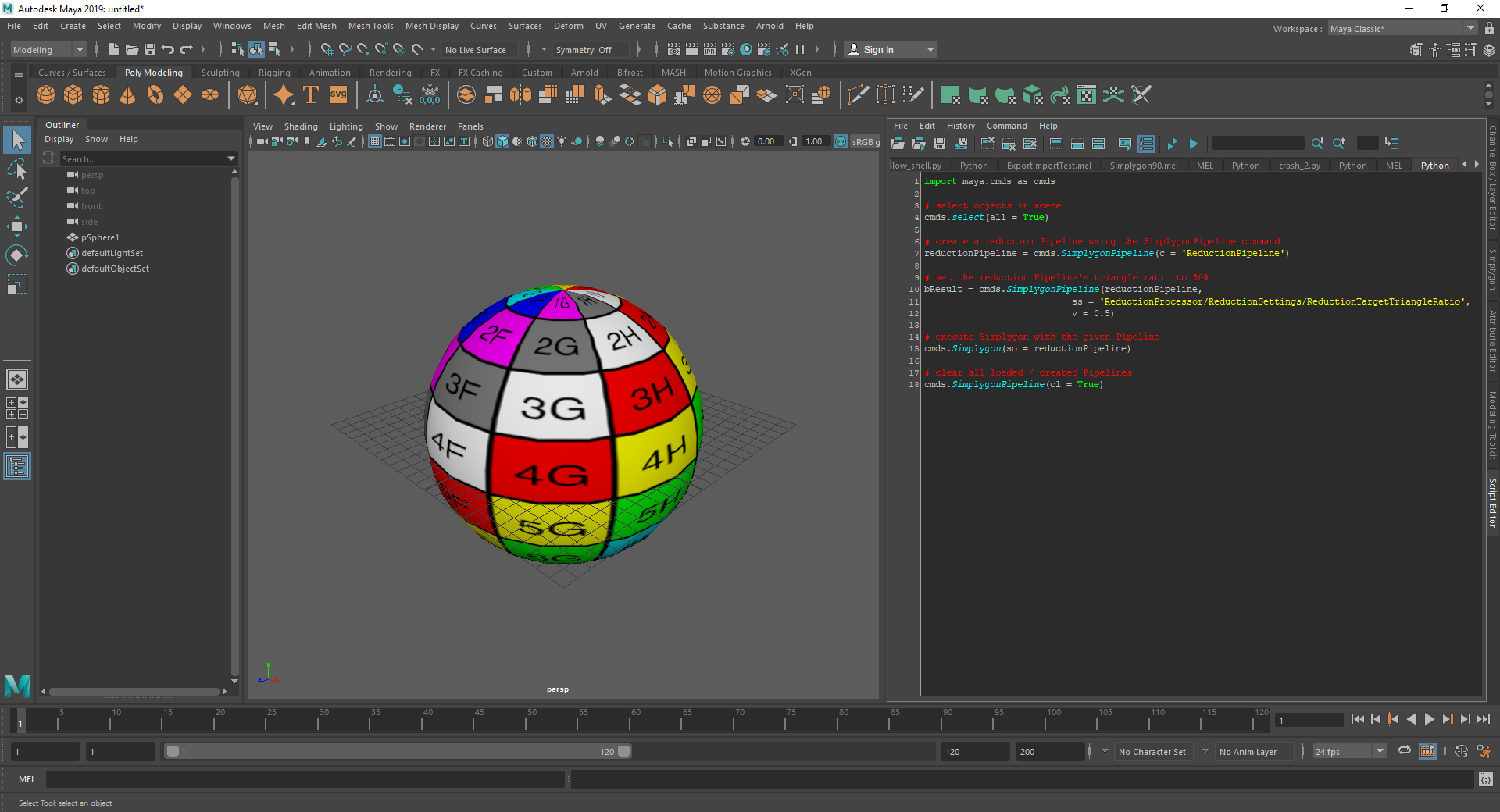
Accessing Simplygon UI
The Simplygon UI exposes a set of functions that can be accessed through script.
Function table:
Function | Argument | Description |
---|---|---|
LoadPipelineFromFile | string filePath | Loads specified pipeline into Simplygon UI |
SavePipelineToFile | string filePath | Saves pipeline loaded in the Simplygon UI to specified file |
Script examples:
// load pipeline
SimplygonUI -LoadPipelineFromFile "D:/Pipelines/ReductionPipeline.json";
// save pipeline
SimplygonUI -SavePipelineToFile "D:/Pipelines/ReductionPipeline_Copy.json";
import maya.cmds as cmds
# load pipeline
cmds.SimplygonUI('-LoadPipelineFromFile', 'D:/Pipelines/ReductionPipeline.json')
# save pipeline
cmds.SimplygonUI('-SavePipelineToFile', 'D:/Pipelines/ReductionPipeline_Copy.json')
IMPORTANT NOTE - Scripting and UI modules
The Simplygon Maya scripting - and UI modules are separate plug-ins. The scripting module encapsulates the import and export logic as well as scripting capabilities. The UI module is a separate layer that communicates with the scripting layer. Communication between these modules require both modules to be loaded.
Dependencies:
- The scripting module can be used without other dependencies (through script functions)
- The UI module must be used in combination with the scripting module
Next steps
Get to know how to use the Simplygon Maya plug-in: